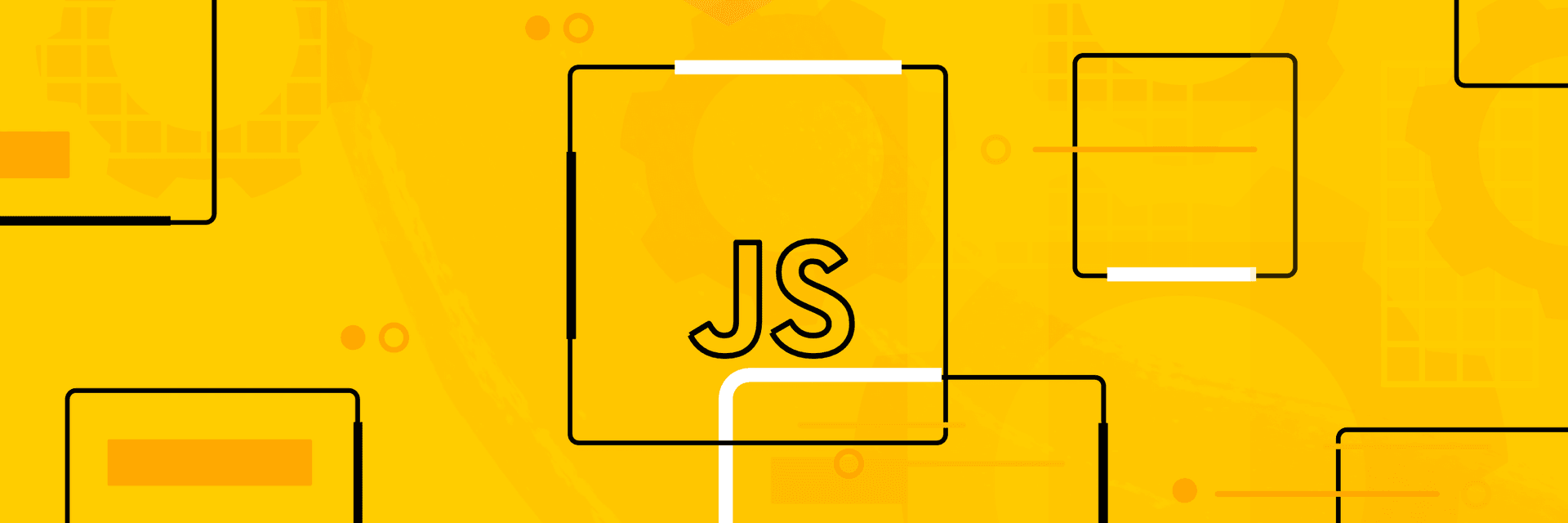
JavaScript tips and tricks
JavaScript was originally named JavaScript in hopes that people would use it due to the affiliation of the name Java
- but it has nothing to do with Java.
Netscape was the one who first submitted Javascript to ECMA International for standardisation (ECMA is an organisation that creates standards for information). As a result, a new language standard is born called what we know as ECMAScript
In very layman terms, ECMAScript is a standard while Javascript is considered to be the most popular implementation of said standard.
There are many revisions of ECMAScript, with the latest one generally referred to as Javascript ES11
Each revision brings new updates and features to the language, we're going to cover a few handy features that ES has to offer. We're going to do this by firing quick "interview style" questions, and giving quick "interview style" responses.
What is the difference from .call, .apply, .bind?
call
method will execute a function with a given this
value and individual arguments, an example of using call
would be:
javascriptvar car = { registrationNumber: 'GZC955', brand: 'Mitsubishi' } function displayDetails(ownerName) { console.log(`${ownerName} this is your car ${this.registrationNumber} ${this.brand}`); } displayDetails.call(car, 'Mike'); // Mike this is your car GZC955 Mitsubishi
`apply
does the same, but instead of individual arguments, an array is passed in, our examples for call
and apply
are almost the same, except you would pass Mike
in as an array instead:
javascriptdisplayDetails.apply(car, ['Mike']); // Mike this is your car GZC955 Mitsubishi
Becuase apply
allows you to pass any iterable, it means you can pass on the arguments
variable from within a function:
jsfunction createDisplayDetails(car) { return function() { displayDetails.apply(car, arguments); } } const displayForCar = createDisplayDetails(car); displayForCar("Mike")
bind
is a little bit different in that it will return a new function instead of executing it like call
and apply
, an example why you would use bind
is:
javascriptvar car = { registrationNumber: 'GZC955', brand: 'Mitsubishi', displayDetails: function() { console.log(`${this.registrationNumber} ${this.brand}`); } } car.displayDetails(); // GZC955 Mitsubishi
This will display GZC955 Mitsubishi
which is correct - but what if you want to attach that to a variable?
If you tried:
javascriptlet myCar = car.displayDetails; myCar(); // undefined undefined
This is a proper example of context loss - to fix this, you can use bind
!
javascriptlet myCar = car.displayDetails.bind(car); myCar(); // GZC955 Mitsubishi
What does this mean in Javascript?
this
is a reference to the bound context, it could be the object that a function belongs to, the global object (globalThis
), or the provided thisArg
to fn.call(thisArg)
, fn.bind(thisArg)
, or fn.apply(thisArg)
.
What is the difference between .map and .forEach?
The difference is that a map
iterates over an array and returns the result, a quick and easy map
example:
javascriptlet array = [1, 4, 9, 16]; let map = array.map(item => item * 2); // we're just multiplying the original array by 2 console.log(map); // [ 4, 16, 38, 320, 24 ]
forEach
only iterates over an array, returning undefined
once complete. a forEach
example:
javascriptlet array = ["a", "b", "c", "d", "e", 10, 5]; array.forEach(item => console.log(item)); /* console.log(item) will log: a b c d e 10 5 */
What is a closure?
A closure in Javascript is a lexical scope.
In our example the inner
function that has access to the variables defined in the outer
functions scope:
javascriptfunction outer() { let a = 10; function inner() { console.log(a * 2); } return inner(); } outer(); // 20
What is the Javascript Event Loop?
This is not strictly related to JavaScript as ES (ES is simply short for ECMAScript.) - however it is the runtime that defines and implements it - Javascript doesn't know anything about it and doesn't care. However it's useful to know if you're a developer because it helps you understand how things work in the background and what actually allows your code to run.
This is a concurrency model based on an "event loop" - which is extremely different from other languages.
JavaScript always runs single threaded. There is always only one thing happening at a time. This is actually a good thing as it will help simplify the way you develop Javascript apps.
The event loop is what allows JavaScript to use callbacks and promises. The event loop continuously checks the call stack which is a LIFO (Last In, First Out) queue to determine if there's any function that needs to run.
While this loop is happening, it will add any function call it finds to the call stack and will execute each one in order.
This keeps going until the call stack is empty. (You may be familiar with this if you've ever seen an error log on Chrome DevTools Console)
An example loop:
javascriptlet dogSays = () => console.log("woof"); let catSays = () => console.log("meow"); const main = () => { console.log("ran main"); dogSays(); catSays(); }; main();
If you ran the above code you'll see that:
ran main
woof
meow
Were the results. This is because the event loop on ever iteration looks if there's something in the call stack and executes it until it's empty.
It went like this:
main()
main()
=>console.log('ran main')
main()
main()
=>dogSays()
main()
=>dogSays()
=>console.log('woof')
main()
=>dogSays()
main()
main()
=>catSays()
main()
=>catSays()
=>console.log('meow')
main()
=>catSays()
main()
- empty
The event loop got its name because of how it's usually implemented which usually resembles something like:
javascriptwhile (queue.waitForMessage()) { queue.processNextMessage(); }
queue.waitForMessage()
will wait in sync for a message to arrive if there is none.
An extremely interesting property of the event loop is that in Javascript, unlike a lot of other development languages, it never "blocks". Handling I/O (Input/Output) is typically performed by way of events and callbacks so when the application is waiting for something specific (like an XHR request to return), it can still process other things like user input.
You can read more about this over on MDN
Javascript Prototype Functions you should know
Array.prototype.every
.every()
method is used to test whether all the elements in the array pass the test implemented by a provider function.
Take the example below.
javascriptfunction isBelowThreshold(currentValue) { return currentValue < 40; } let array = [1, 30, 39, 29, 10, 13]; console.log(array.every(isBelowThreshold)); // true
Array.prototype.some
The .some()
method tests whether at least one element inside the array passes a test implemented by the provided function (so very similar to every
)
Take the example below:
javascriptlet array = [1, 2, 3, 4, 5]; let even = function(element) { return element % 2 === 0; }; console.log(array.some(even)); // true
Array.prototype.includes
.includes()
is a favourite of mine - this method will check if an array includes the item passed in.
javascriptlet array = [1, 2, 3, 4, 5]; arr.includes(2); // true arr.includes(10); // false
Array.prototype.filter
The .filter()
method will create a new array with only the elements that have passed the condition inside the provided function:
javascriptlet array = [1, 2, 3, 4, 5, 6, 7, 100]; let filtered = array.filter(num => num > 4); console.log(filtered); // [ 5, 6, 7, 100 ] console.log(array); // [ 1, 2, 3, 4, 5, 6, 7, 100 ]
Array.prototype.reduce
The .reduce()
method is pretty awesome. It's not used nearly enough as it should be! This method will apply a function against an accumulator in each element in said array to reduce it to a single value, you can also provide an initial value (if you leave this out, the first value of the array will be used
javascriptlet money = [13.37, 21.85, 16.5, 5, 12, 242]; let initialValue = 0; let sum = money.reduce((total, amount) => total + amount, initialValue); console.log("Sum: ", sum); // 310.72
Array.from
One function that's rarely used is Array.from()
. This method will create a new shallow-copied Array instance from an array-like or iterable object (like a string):
javascriptlet name = "michael"; let nameArray = Array.from(name); console.log(name); // michael console.log(nameArray); // [ 'm', 'i', 'c', 'h', 'a', 'e', 'l' ]
Array.from
Another one is the Array.of()
method that will create a new Array
instance from a variable number of arguments.
javascriptlet first = Array.of(7); let second = Array.of(1, 2, 3); console.log(first); // [7] console.log(second); // 1, 2, 3
What is event propagation and bubbling?
This one is more related to usage of JavaScript with the HTML DOM, but it is still good information to know!
Bubbling is when events execute in an inner element all the way up through the parent elements.
html<style> body * { margin: 10px; border: 1px solid blue; } </style> <form onclick="alert('form')">FORM <div onclick="alert('div')">DIV <p onclick="alert('p')">P</p> </div> </form>
If you were to click the P
(and inner most element), then you would receive 3 alerts:
p
div
form
It's called bubbling because of bubbles in the water and how they rise.
Event propagation on the other hand, is a way to describe the stack of events that are fired and can be used to stop the bubbling as shown above.
If we needed to stop other events in a stack from bubbling, we can use event.stopPropagation()
within an event handler (which you would add using event.addListener("name", function handler(event) { })
)
What would be the reason to use ES classes?
For object oriented programming (OOP) and not needing to learn prototype chains, ES classes give more of a native OOP developer experience.
What is the virtual DOM?
Firstly, a DOM is a Document Object Model which is an API for HTML and XML documents. It defines the logical structure of documents and the way a document is assessed and manipulated. This API provides a way for JavaScript and other languages to interact with any node to manipulate it.
A node is how everything inside the DOM is represented. The document, HTML elements, and even text.
The Virtual DOM is a programming concept where the representation of a UI is kept in memory and synchronised with the real DOM.
In easier to understand language, a Virtual DOM is a representation of the HTML DOM that can be effeciently traversed and "diff'd" to ensure the DOM isn't manipulated when it isn't needed.