How to efficiently debug JavaScript with Chrome DevTools.
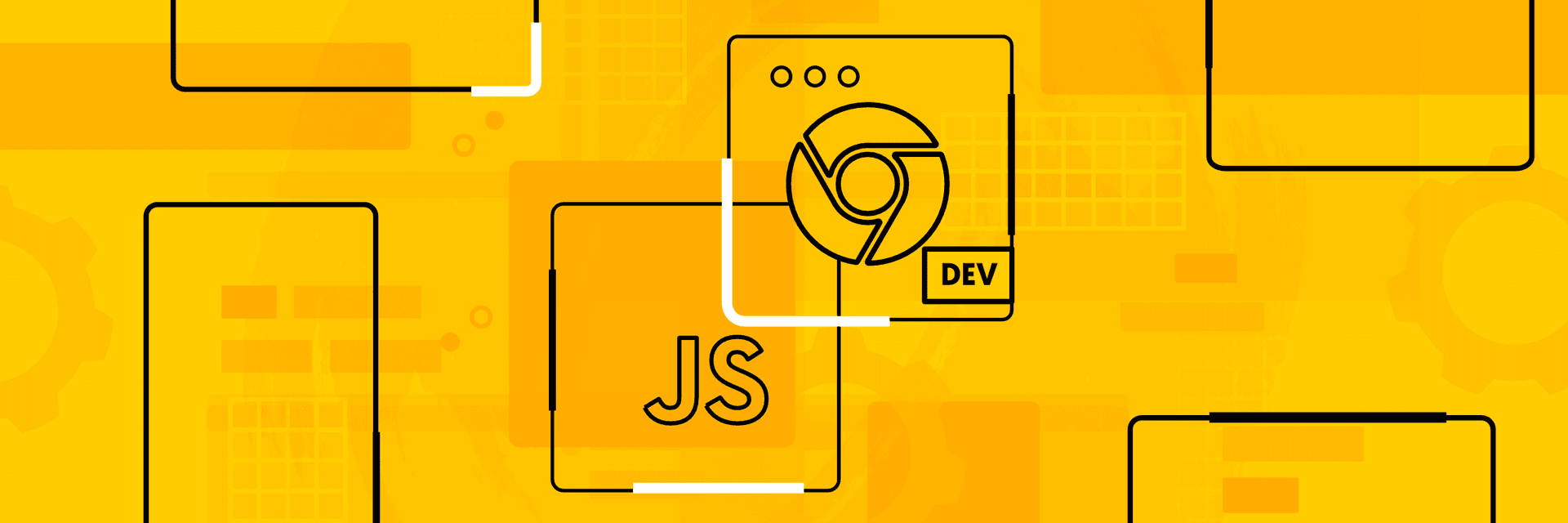
March 31, 2021 | Last updated: January 2, 2024
Table of Contents
Cover image - Chrome DevTools
In this article, you'll learn how to use Chrome DevTools to debug JavaScript code in an efficient manner.
What are Chrome DevTools?
The DevTools are internal developer tools that come with every version of the Google Chrome browser. The tools facilitate code debugging, allowing users to add breakpoints to detect issues and fix them directly in the browser.
DevTools panel overview
The DevTools consist of 8 main panels:
- Elements: View the DOM tree and manipulate HTML/CSS and style attributes
- Console: View and debug your JavaScript code
- Performance: Analyze and optimize the speed of web page or application
- Sources: Debug JavaScript code for syntax errors, set breakpoints, call stack, and inspect variables
- Network: Monitor network, view HTTP requests, and simulate different network conditions
- Application: Inspect localStorage, sessionStorage, cookies, IndexDB, and modify storage data
- Memory: Track memory usage, garbage collection, and memory leaks, and fix related issues
- Security: View SSL certificate details and insecure content warnings
- Lighthouse: Audit your app for performance, accessibility, and SEO
Chrome DevTools sources panels
DevTools panel keyboard shortcuts
To open the DevTools Elements panel, press:
- MacOS:
Command + Option + C
- Linux/Windows:
CTRL + SHIFT + C
To open the DevTools Console panel, press:
- MacOS:
Command + Option + J
- Linux/Windows:
CTRL + SHIFT + J
How to Debug JavaScript with DevTools
The DevTools are basically bread and butter of every developer and don't require much description, so let's get down to some useful strategies that will let you debug your code more efficiently.
1. Add breakpoints
Breakpoints are a powerful debugging feature because they pause code execution, so you can inspect line by line and choose to resume once you're ready. This is especially useful for large source code bases or when it is hard to pinpoint the source of the bug.
Adding a breakpoint
In this example, let's add a line-of-code breakpoint.
Open DevTools and switch to the Sources panel.
In the Page window, select the .js file in which you want to add the breakpoint. The file source code will appear in the middle code editor panel:
Selecting the JavaScript file
- Right-click the desired line in the gutter and select 'Add Breakpoint':
Adding a breakpoint
- Run the function now. In my case, it pauses right before executing the POST request, plus I can see the posted
data
:
Running the function
- Once you confirm that everything is in order, click the resume button to continue script execution.
Resuming script execution
console.log(data)
and reloading the page over and over again.
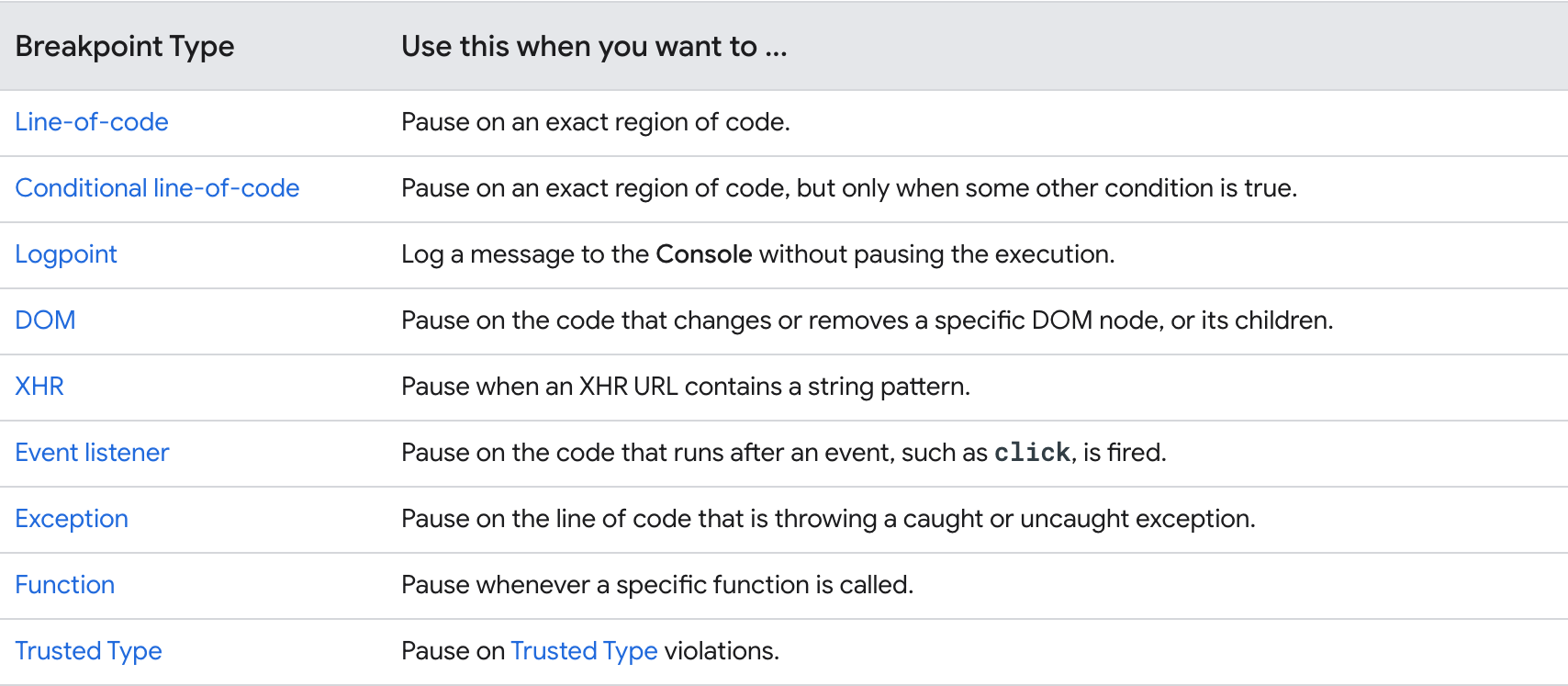
2. View and edit local, closure and global properties
While the app is paused, you can view and edit the local, closure and global properties. Let's assume there's a bug that does not return the correct value for a variable, so you want to check its value at a certain point in the current function.
Once we add the breakpoint, we can expand the 'Scope' panel in the right column of the dev tools and view the variable value. If you want to test the function with other values, you can double-click on the variables to edit.
scope panel
3. Create, save and run snippets
Another efficient strategy is employing snippets. Snippets allow you to easily execute and reuse scripts in any part of your app via the Google's developer tools.
- You can add a snippet by switching to the Snippets tab in the left column.
Adding a new snippet
With the tab open, click +New Snippet and write your code in the middle panel as shown in the image below:
New snippet overview
CMD+S
/ CTRL+S
at any time.
The snippet can be executed in two ways:
- Right-click on the snippet and click 'Run'.
- Press
CMD + Enter
orCTRL + Enter
.
Running a snippet
4. View call stack
When debugging the error, you may want to track the changes to the call stack. To display the functions in the stack, switch to the Sources panel and click the Call Stack line to expand it.
Call stack panel
5. Blackbox
When debugging, you probably want to exclude some scripts from running, such as third-party libraries, or scripts that you know are unrelated to the error.
Instead of commenting them out in your source code line by line, you can blackbox them on DevTools. To do so, click on the script file you want to ignore in the left panel on the Sources tab. Then, right-click on the middle panel and select 'Add script to ignore list':
Blackboxing a script
From now on, the script will no longer run, which helps narrow down and eliminate the cause of the error or bug.
Conclusion
The possibilities that Chrome DevTools allow for are basically endless. I highly recommend exploring the provided links in the Resources section below to delve further into these features.
Once you become proficient with the toolset, you will enhance your debugging skills and leave behind the days of relying on the console.log()
grind.
Thank you for taking your time to read this tutorial. If you found the article helpful, please consider sharing it. Cheers!
Additional resources
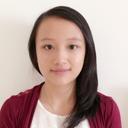
Victoria Lo
Solutions Engineer @ PayPal
Victoria Lo is currently a Solutions Engineer at PayPal. Her background is in full-stack software/web development. She loves to share her knowledge on programming and give advice for new developers on her blog. Besides being passionate about blogging and software, she loves to read books, play video games and collect quotes.
Read similar articles
Building a Web App with Angular and Bootstrap
Check out our tutorial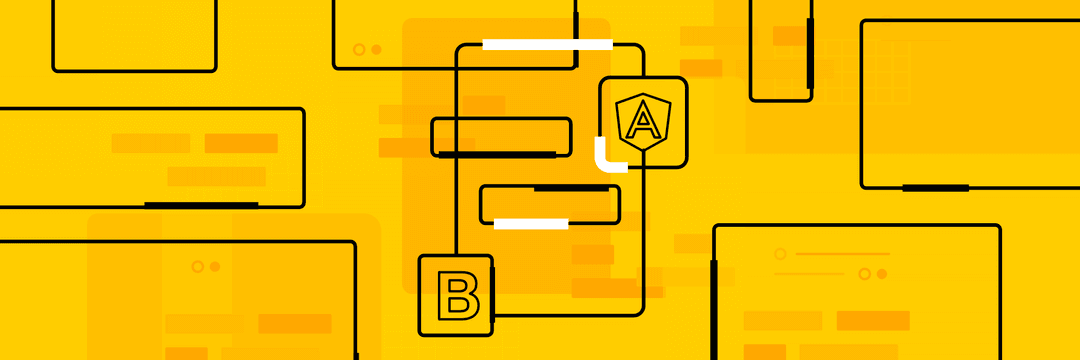
Let's play bingo with JavaScript!
Check out our tutorial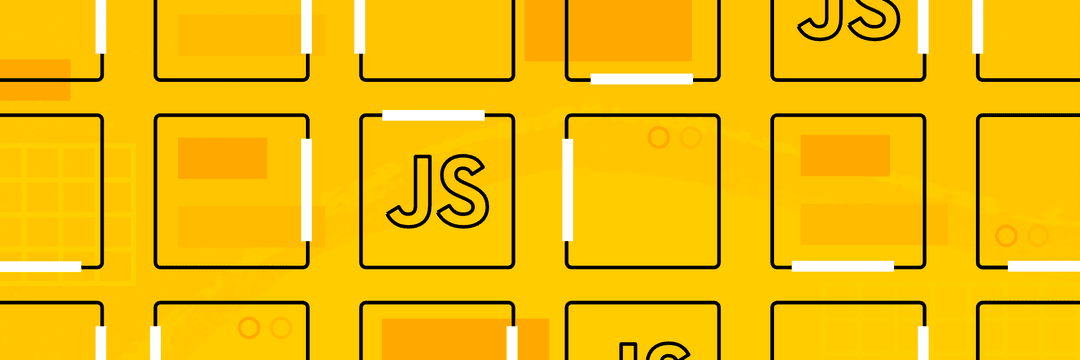
GraphQL Subscriptions - Core Concepts
Check out our tutorial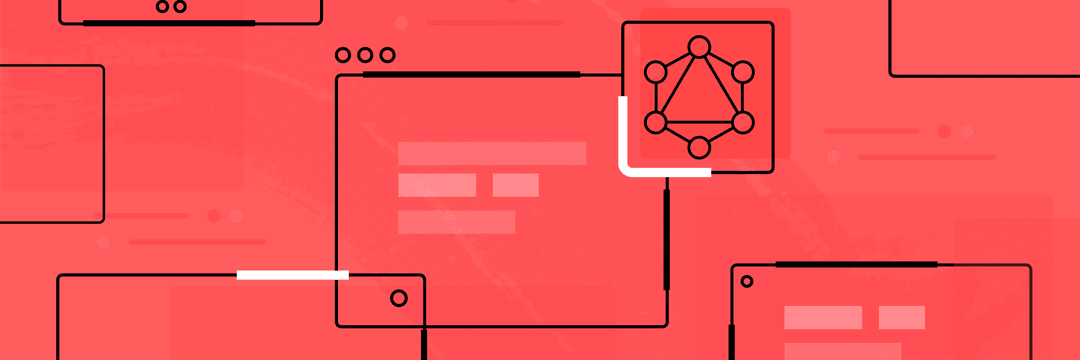