Vue directives
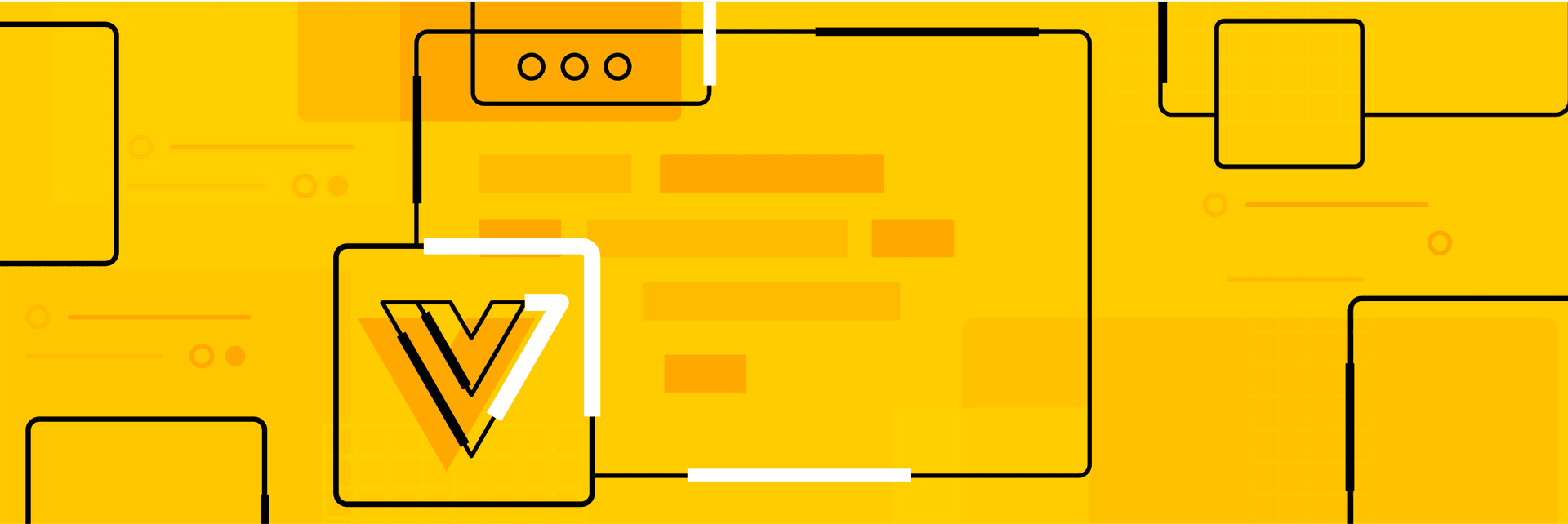
December 8, 2020
This article is part 5 of the series on Vue.js. Check out the other articles:
In this article we will learn about the tool that is commonly used across every Vue application and can save you a lot of time - Vue directives.
What exactly are Vue directives?
We've seen some of Vue directives previously but never explained the concept in depth. Vue directive is just a piece javaScript code binded to a certain DOM element or component that does something with this element (i.e hide it on some conditions). They look similar to HTML attributes but are usually prefixed with a v
letter. A directive look more or less like this:
<dom-element v-directive-name=”directiveValue”/>
Main difference between raw HTML attribute and directive is a fact that it's value is treated as JavaScript code (so it works the way same as we have seen with v-bind). It's important to mention that directive applied without any value evaluates to true
by default
<dom-element v-directive-name /> // value of this directive will be `true`
<dom-element v-directive-name=”someProperty’ /> // someProperty is a data property of Vue instance
Let's explore the most popular Vue directives that are commonly used in almost every Vue application:
V-show
This directive is used to visually hide a certain DOM element. It just a adds display: none
CSS property to applied element when it's value is false
and does nothing otherwise.
A good example of v-show usage is showing/hiding a modal window.
<modal-window v-show=”isModalVisible” />
V-if
V-if works the same way as v-show except instead of visually hiding element it completely removes it from the DOM. It's important to be aware of implications of such process. Even though both component below will be hidden only the the first one will fire it's lifecycle hooks. The second one's hooks will execute only after isModalVisible value will be set to true
(and rerun them each time value changes from false to true again).
<modal-window v-show=”isModalVisible” />
<modal-window v-if=”isModalVisible” />
V-else
You can use v-else in combination with v-if to provide a fallback for conditional statements.
This code:
<truth-only-component v-if=”isTruth” />
<false-only-component v-else />
Is just a nicer way of writing:
<truth-only-component v-if=”isTruth” />
<false-only-component v-if=”!isTruth” />
You can use this directive to make your code cleaner and easier to understand.
Here you can find working example of v-if and v-show usage. Play with it and observe the console..
V-model
We want to use v-model whenever we have some forms on our website. This directive binds data properties to input elements which means:
- whenever value of the input changes data property changes
- Whenever data property changes input property changes
This nice mechanism is called two-way data binding because no matter where we will mutate the data (input/script) the change is immediately reflected on the other "side".
The value passed to v-model is always a data property:
<input name=”name” v-model=”user.name”>
We can use v-model to bind data properties to:
- inputs
- textareas
- checkboxes
- radio groups
- selects
You can find great examples for each of this use cases in Vue documentation and here you can play with working example.
V-for
This directive is commonly used to iterate through Arrays and Objects. Element that it was applied to will be repeated in DOM for every Arrays item.
Let's say we have a list of dogs like this:
data () {
return {
dogs: [“Alpha”,”Bravo”,”Charlie”]
}
}
Now to display them in a nice HTML list we just need to pass this object into v-for directive:
<ul>
<li v-for=”dog in dogs”>{{ dog }}<li>
<ul>
<li> element will be repeated 3 times. It's important to know that "dog" is a variable that holds value of currently rendered list element so it changes on every iteration.
This is how the output HTML will look like:
<ul>
<li>Alpha<li>
<li>Bravo<li>
<li>Charlie<li>
<ul>
First argument of v-for can actually be a tuple and along with the list item return it's number (starting from 0). The code below
<ul>
<li v-for=”(dog, id) in dogs”>{{ id }} {{ dog }}<li>
<ul>
Will generate this HTML:
<ul>
<li>0 Alpha<li>
<li>1 Bravo<li>
<li>2 Charlie<li>
<ul>
Sometimes when we are dealing with complex lists we would like to modify some of it's values. Usually such change triggers re-rendering of whole list. To prevent it we can pass a unique key identifier for each of it's items. If the key remains the same for some elements of the list Vue will reuse and reorder them instead of re-rendering the whole list.
<div v-for="item in items" v-bind:key="item.id">
<!-- content -->
</div>
We can use v-for to iterate through objects the same way as through Arrays. When used as a tuple the second argument will be Object's property name.
// in component instance
data () {
return {
dog: {
name: “Buddy”,
age: 2
}
}
}
// in template
<div v-for="(value, name) in dogs">
{{ name }}: {{ value }}
</div>
The code above will generate this HTML:
<div>name: Buddy</div>
<div>age: 2</div>
You can find a working example here.
Summary
- Vue directives can be used to bind certain behavior into DOM element or component.
- V-show is used to visually hide certain element with "display: block" CSS property.
- V-if works similar to v-show except it's detaching certain element from the DOM completely which implies that it's lifecycle hooks won't be executed.
- You can use v-else instead of two v-ifs to make your code more readable.
- You can use v-model when creating interactive forms to bind data properties to form elements like input or checkbox.
- V-for let's you easily iterate through objects and Arrays in your templates.
Next in series: Navigating through Vue applications - Vue Router fundamentals
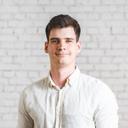
Filip Rakowski
CTO & Co-founder @ Vue Storefront
I'm a Software Developer specialized in JavaScript and modern frontend technologies. Building open source products and communities around them is what I love and do best. I'm successfully running 2 big scale open source projects - Vue Storefront and storefront UI and contribute to VuePress as it's core team member. I'm also a very active member of Vue.js community. I am official community partner of this framework, Wrocław Vue community leader and speaker on international Vue and JavaScript conferences. Despite other activities I love sharing knowledge and teaching programming.
Read similar articles
Building a Web App with Angular and Bootstrap
Check out our tutorial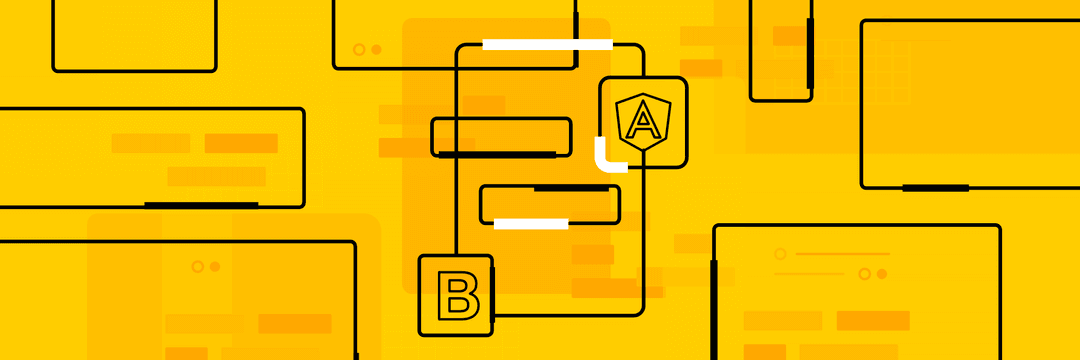
How to efficiently debug JavaScript with Chrome DevTools.
Check out our tutorial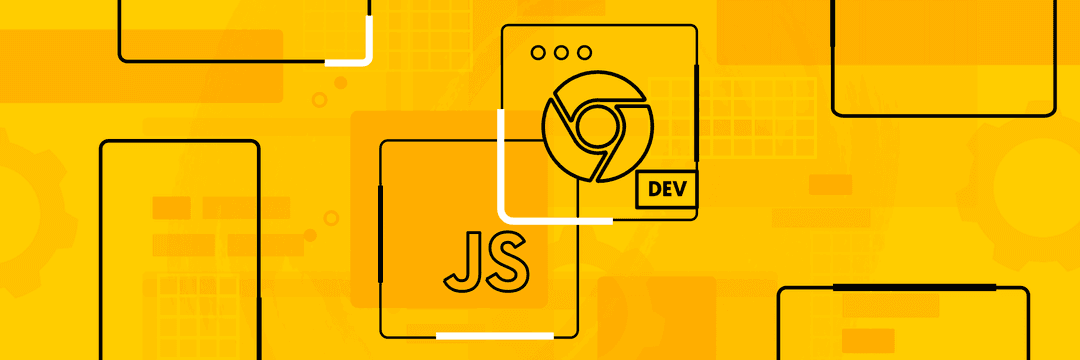
React vs. Vue.js: What's the best in 2021
Check out our tutorial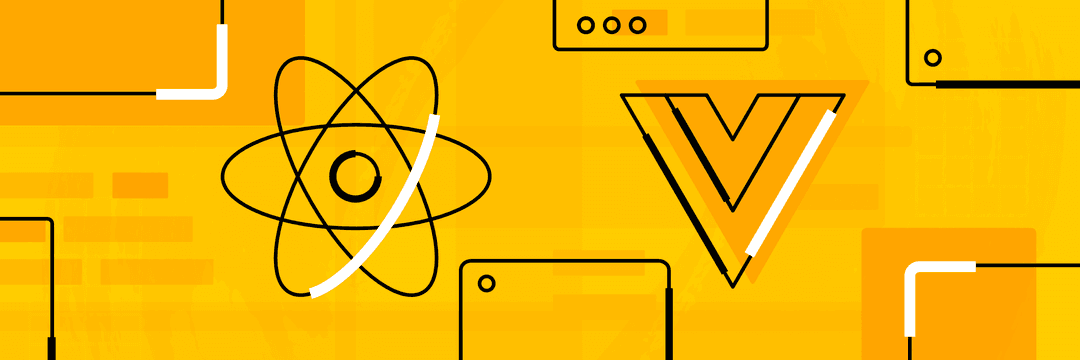