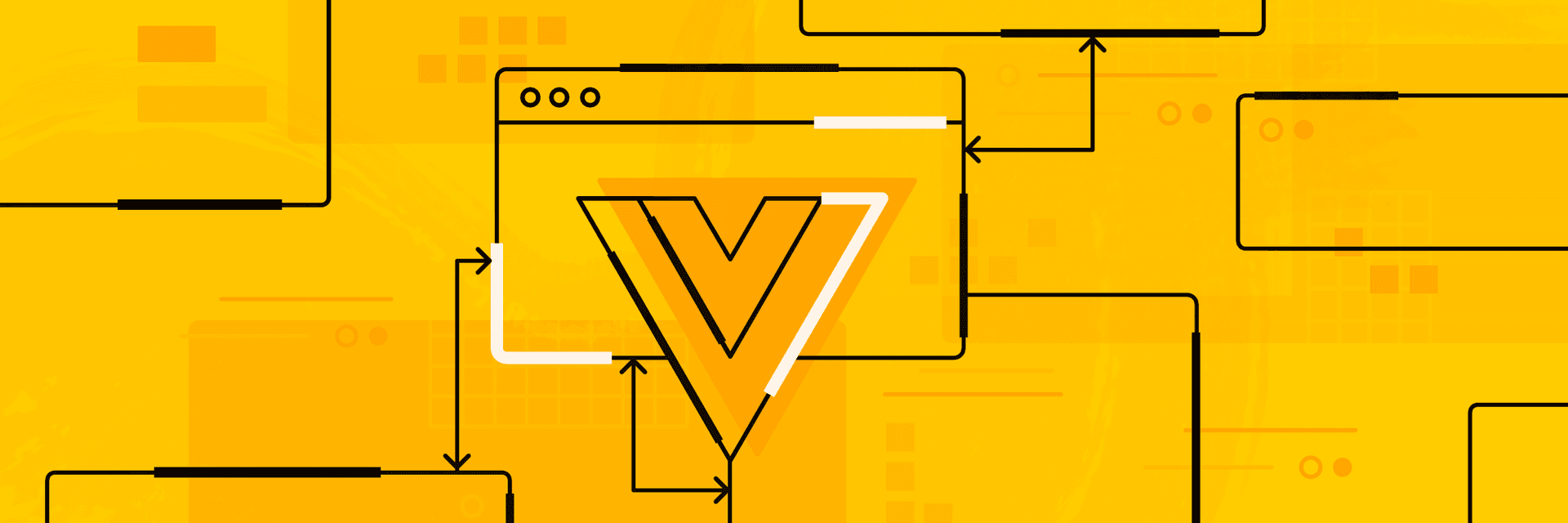
Vue instance and its properties
This article is part 2 of the series on Vue.js. Check out the other articles:
In the previous article we had a brief overview of what Vue is and how it works on a very abstract level. Now it's time to dig deeper and write some real code.
What is Vue instance?
Every component that you will ever create is a Vue instance. It's nothing else but a single instance of Vue object which implies that our app is in fact built from a number of micro applications. It's also a good guidance to understand how to think about components. Try to make them encapsulated, standalone and not tied to other components - just like a separate applications.
Let's start with the basics and understand how every Vue instance works. By learning it's most common properties (also called options) we will learn how to make use of it.
Showing the content - el, template and render instance properties
One of the most important properties of Vue instance is el. The DOM element that's selector will be passed to this property will be a place where our Vue application will live.
Let's say we have an index.html file with a div inside of it. Let's give it id app.
html<div id=”app”></div>
We want our Vue application to be rendered inside of it. What it actually means? It means that everything inside of this div will be rendered by Vue engine and it will have a full control over this DOM element but everything outside will remain unchanged. This feature lets Vue play very well with other code. You can make a small Vue application inside any other app just by passing a proper DOM selector. The below code is everything that's needed to bind a DOM element to a Vue instance.
defaultnew Vue({ el: ‘#app’ )}
Once it's binded we can either directly pass a template to render:
defaultnew Vue({ el: ‘#app’, template: ‘<div>Hello from Vue instance!</div>’ )}
...or pass a component to so called render function:
defaultnew Vue({ el: ‘#app’, render: h => h(RootComponent) )}
The h
parameter is actually a common shortcut for 'createElement` method which is responsible for creating and rendering new DOM node with passed component. In this case the render function will take the div with id app and render a component represented by RootComponent object inside of it.
Here we can find a working example.
It's important to remember that el property should be used only in a Root component. When you think about this it makes a perfect sense since our app is rendered only in one place.
Displaying and modifying data - data and methods properties
defaultdata () { return { message1: ‘value1’, message2: ‘value2’ } }
We have seen a data property in a previous lesson. It's nothing else but a state of your component. You can display data properties directly in the template and refer them in Vue instance via this.propertyName.
All of the properties from object returned by data are added to Vue reactivity system which means whenever they're changed all of their occurrences will change aswell.
The methods instance property, as name suggests, is a container for methods associated to a particular component. You can use and change the data properties there. For example if we would like to change the value of a message1 property we can do this by adding changeMessage method to our component:
defaultmethods : { changeMessage () { this.message1 = ‘new value’ } }
We accessed the massage1 property from this object referencing to our component instance and overrode it's value. Whenever we used this property the value will also change thanks to Vue reactivity system. It's important to remember that we can access methods in the same way we are accessing data properties so changeMessage method can be accessed within Vue instance via this.changeMessage.
Here you can find working example with usage of methods instance property.
Click on a button in the example. You will notice that value of msg property in a template immediately changed. I strongly suggest you to play with this code and create your own variables and methods to better understand this concept. The @ that you will find in a template is a shorthand for v-bind directive that we learned in the previous article.
Formatting, grouping and filtering data - computed properties
Let's say we have a student data in our components state
defaultdata () { return { student: { name: ‘John’, surname: ‘Doe’, grades: [ 3, 4, 5 ], age: 22 } }
Since we know that we can use JavaScript inside mustache interpolation ( {{ }} ) in our templates displaying full information about given student could look like this:
default{{ student.name + ‘ ‘ + student.surname + ‘ age: ‘ + student.age + ‘ ‘ + student.grades }}
Now imagine that we want to make more complex data operations like displaying the average grade. Putting business logic into our templates not only makes it less readable but also violates separation of concerns rules. This is where computed properties can help us!
Computed properties are designed exactly for this case. Instead of putting the logic directly in our template we can create a computed property that returns the data in the format that we want. The great thing about computed properties is that the're also part of Vue reactivity system which means that if any of the displayed values will change the computed property will also change. Above example can be rewritten to computed property like this:
defaultcomputed: { studentInfo () { return this.student.name + ‘ ‘ + this.student.surname + ‘ age: ‘ + this.student.age + ‘ ‘ + this.student.grades }
We can display computed properties in the template the same way we are displaying a raw state properties. So instead of the very long expression we can simply write this:
default{{ studentInfo }}
Much more elegant. Isn't it?
You can find the working code here. Try to change values of the student object and see what happens. Play with the code if you think you still don't understand how it works.
Summary
In this article we learned the fundamentals of Vue components;
- Every Vue components is an instance of Vue object
- el property mounts the Vue application on a given DOM element
- render property renders passed root component (it can do much more but for now this knowledge is just enough)
- object returned by data property is a state of our component
- methods property contains functions that can modify the state
- computed properties are used to display state property (or properties) in a certain format