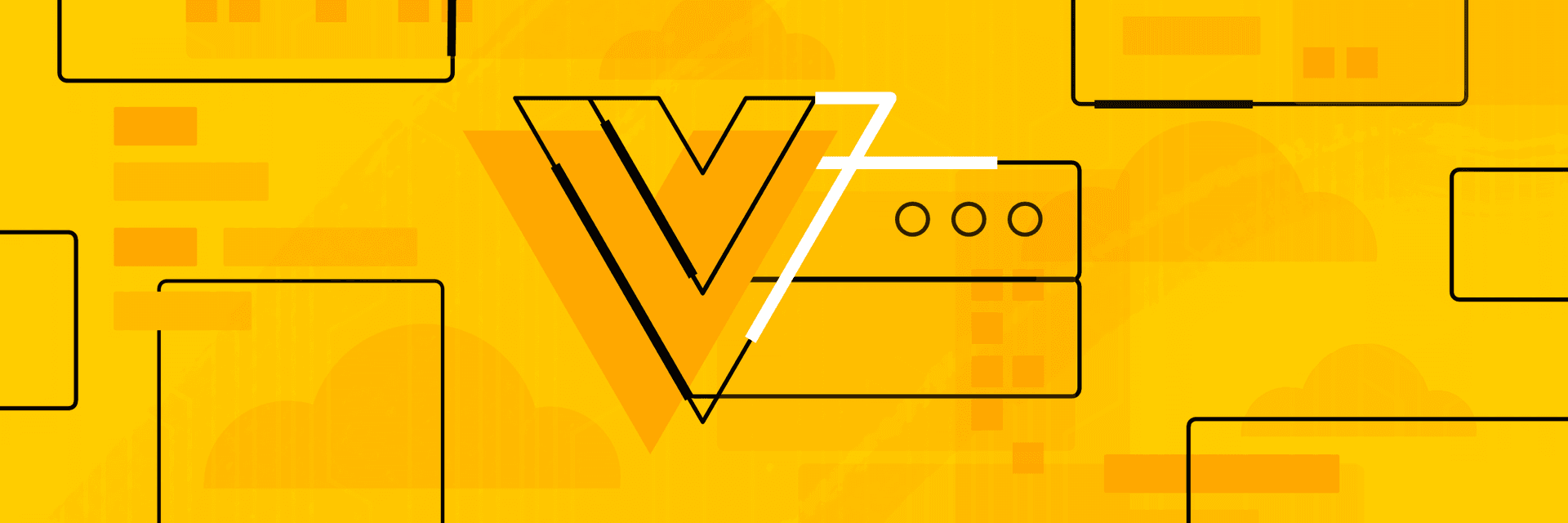
Navigating through Vue applications - Vue Router fundamentals
This article is part 6 of the series on Vue.js. Check out the previous articles:
- Part 1: Introduction to Vue - key concepts and why it's so awesome
- Part 2: Vue instance and its properties
- Part 3: Communicating between Vue components
- Part 4: Vue - Lifecycle hooks
- Part 5: Vue directives
All that we already learned was oriented around creating very simple applications with single view. Even though modern JavaScript apps are called Single Page Apps it doesn't really mean that they contain only one view. This term refers to the fact that only parts of the UI are refreshed which prevents full page reloads and provides a better user experience.
In this article we will explore Vue Router - a plugin that lets you create a Single Page application with multiple views.
What is a route?
A single route is usually a component associated with certain URL. If we would like to display different content on mydomain.com/about and mydomain.com/contact we should create a route for each URL and associate them with a component of our choice. As simple as that.
Vue plugins
Vue Router is a Vue plugin. We havn't touched this concept before but plugins in Vue are the same things as plugins in any other piece of software. They could enrich your application with additional functionality. As we can read in Vue docs "Plugins usually add global-level functionality to Vue. " and this is a best description of what they do.
To install a plugin simply use "use()" function of Vue object and pass the plugin object to it. For example Vue.use(VueRouter).
Installing Vue Router
To add Vue Router plugin to our application we need to follow 3 very easy steps:
- Install the plugin
In command line interface on the root of your app run "npm install --save vue-router" script. It will install Vue Router as new dependency of your project.
- Import Vue Router plugin and register it
Just use Vue.use() method In your JavaScript entry point (usually it's a main.js). It's important to do this before instantiating root Vue instance. Otherwise plugin will not work properly
defaultimport Vue from “vue” import VueRouter from “vue-router” Vue.use(VueRouter)
- In the same file create new Vue Router instance and pass it to root Vue instance.
defaultconst router = new VueRouter() const app = new Vue({ router render: h => h(App) )}.$mount(“#app”)
And that's all! You have a working Vue Router registered in your application! This process is similarly simple for almost every Vue plugin.
Even though we installed Vue Router it's not doing anything meaningful yet. Let's add some routes!
Route object
Vue Router instance that we've just created accepts object as a parameter (similar to Vue instance). The most important property of this object is routes which accepts array of so called route objects. Route object is nothing else but a JavaScript object representation of single route in your application.
Let's see how very basic router could look like:
defaultImport HomePage from “/HomePage.vue “ Import ContactPage from “/ContactPage.vue “ Import PageNotFound from “/PageNotFound.vue” const routes = [ { path: “/”, component: HomePage } { path: “/contact”, component: ContactPage }, { path: ”*”, component: PageNotFound } ] const router = new VueRouter({ routes, mode: “history” })
It's pretty self-descriptive isn't it? Essentially we are just matching Vue components with URLs. In that case HomePage component will be treated as entry route of our application (so it will be displayed when someone entersmydomain.com/) and ContactPage will be displayed after enteringmydomain.com/contact.
Setting path property as "*" will match every other route that wasn't already specified (likemydomain.com/about). It's a good practice to display a dedicated 404 page for those routes and so we did in this example.
It's important to remember about setting mode property value to "history". Otherwise every URL will be prefixed with hash (for examplemydomain.com/#/contact). It's called a hash mode and is a default value of mode property.
Router View
We know how to create routes that display different components under certain URLs but where exactly those components are rendered?
When used as a plugin Vue Router registers a globally available component called <router-view />. This is where our route components will be rendered. The content of <router-view /> will be replaced with a proper route component (so it’s a ContactPage.vue for /about route etc).
<router-view /> component is usually placed along with repetitive elements of the UI that are persisted in every route (usually a header and footer) like in below example:
default<div id=”app”> <AppHeader /> <router-view /> <AppFooter /> </div>
Router link
We know almost everything to use a full power of Vue Router. The only missing part is navigation. Using tag directly will end up with full page reloads which isn’t what we want in our Single Page App. What we need is a reload of <router-view> component. To fulfill this need Vue Router provides us with another component - <router-link>.
Usage is extremely simple. We are passing a path (same as in routes object) to a given route and <router-link> takes care of <router-view> refresh along with URL change. Everything wrapped by <router-link> will become a link the same way as it works with tag.
Let’s say we want to put a navigation in
default<nav> <ul> <router-link to=„/„><li>Home</li></router-link> <router-link to=„/contact„><li>Contact</li></router-link> </ul> </nav>
And that's all! This is all you need to know to create a routable Vue application!
Here you can find working example of code from this article. Play with it and try to add more routes.
Summary
- Vue Router is a Vue plugin that let you handle URL-based navigation on your Single Page Apps
- Vue plugins "usually add global-level functionality to Vue.". You can register them with Vue.use()
- Every Vue Router instance should contain routes object with component-URL pairs.
- <router-view> component is replaced with route component depending on URL
- <router-link> will let you navigate through your application without full page reloads