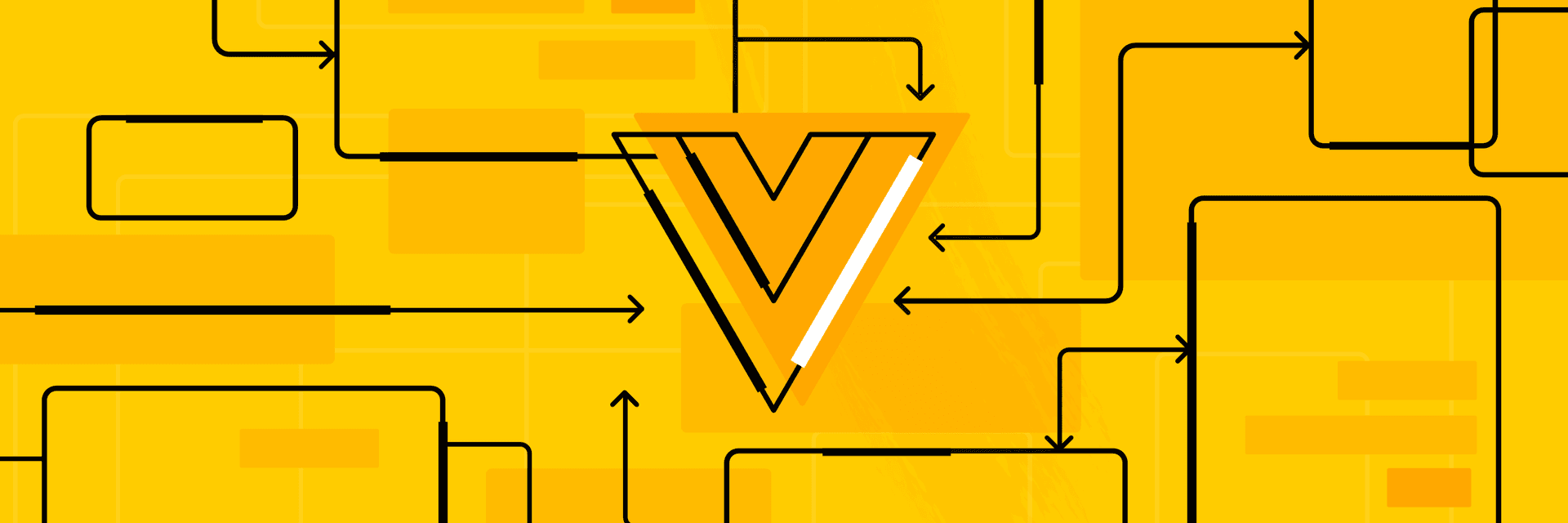
Vue - Lifecycle hooks
This article is part 4 of the series on Vue.js. Check out the other articles:
You know the most important Vue instance properties and how to make components communicate with each other. It’s almost enough to build complex single-route applications. In this article we will go through one of the last missing pieces of Vue fundamentals.
Component lifecycle hooks
When browser engine is executing code responsible for Vue component creation certain initialization steps are performed until this process is complete.
First the component object needs to be initialized - events, props and data properties are bonded to reactivity system. Then template is compiled into a render function which is a JavaScript representation of HTML-like markup we write in a template
property. Once the instance is ready and template is compiled the component is getting mounted in a certain place of the DOM tree. At this point we can say that it’s ready.
Right before component destroyed (for example when we leave the page) it starts to clean up after itself. Child components are destroyed, event listeners are unregistered and watchers are removed so there is nothing left in the memory.
It would be really useful if we would be able to perform some actions on certain steps of component initialization like fetching the API before component is rendered so we can display the data faster. This is exactly what Vue lifecycle hooks are made for.
Vue exposes hooks to perform certain actions on any almost every step of component creation. Let’s learn about them in the order:
- beforeCreate
At this point component data is not available yet. This lifecycle hook is not very useful in standard use cases.
We can access this lifecycle hook by simply adding function with it’s name to our instance properties
defaultbeforeCreate () { console.log(“Hello from beforeCreate. I can’t do much right now’”) }
- Created
At this point we have access to components data but it’s not rendered in the DOM tree yet. This is one of the most useful lifecycle hooks and a perfect place to fetch some external resources.
defaultcreated () { console.log(„Hello from created. Now we can display component properties", this.someProperty) }
- beforeMount
Template is translated to render function and component is ready to be mounted in DOM.
defaultbeforeMount () { console.log("Hello from beforeMount. I’m ready to be mounted!”) }
- Mounted
defaultmounted () { console.log("Hello from mounted! I’m ready now”) }
At this point component is visible and instantiation process is ready.
- beforeDestroy
This hook is fired right before component is destroyed. It’s a perfect moment to delete side effects like
defaultbeforeDestroy () { console.log("Hello from beforeDestroy. You can still access my instance but It’s your last chance”) }
- Destroyed
The component instance is not existing anymore and we don’t have access to any of it’s properties. This function is just a callback fired after component is fully flushed.
defaultdestroyed () { console.log(“Bye bye from destroyed. I don’t exist anymore!”) }
We also have access to two additional hooks that are fired only when component is updating itself which usually happens when some of it’s properties is modified.
Those hooks are beforeUpdate and updated
defaultbeforeUpdate () { console.log(“Hello from beforeUpdate!”) } updated () { console.log(“Here is the new value of someProperty”, someProperty) }
Play with this code example to better understand when certain lifecycle hooks are fired and how to make use of them.
A note about Server Side Rendering
You should be aware that only created and beforeCreate hooks are fired on the Server Side. It’s important to know this to avoid memory leaks generated by side effects. An example for such memory leak could be setting up side effects in created hook and removing them in beforeDestroy. Those side effects will be removed only on client side and take more and more memory on the server since beforeDestroy hook is never called there.
Summary
We learned about the initialization process of Vue components and how to hook into almost every moment of their creation and deletion process. It’s important to remember that some of the hooks are called only on client side.