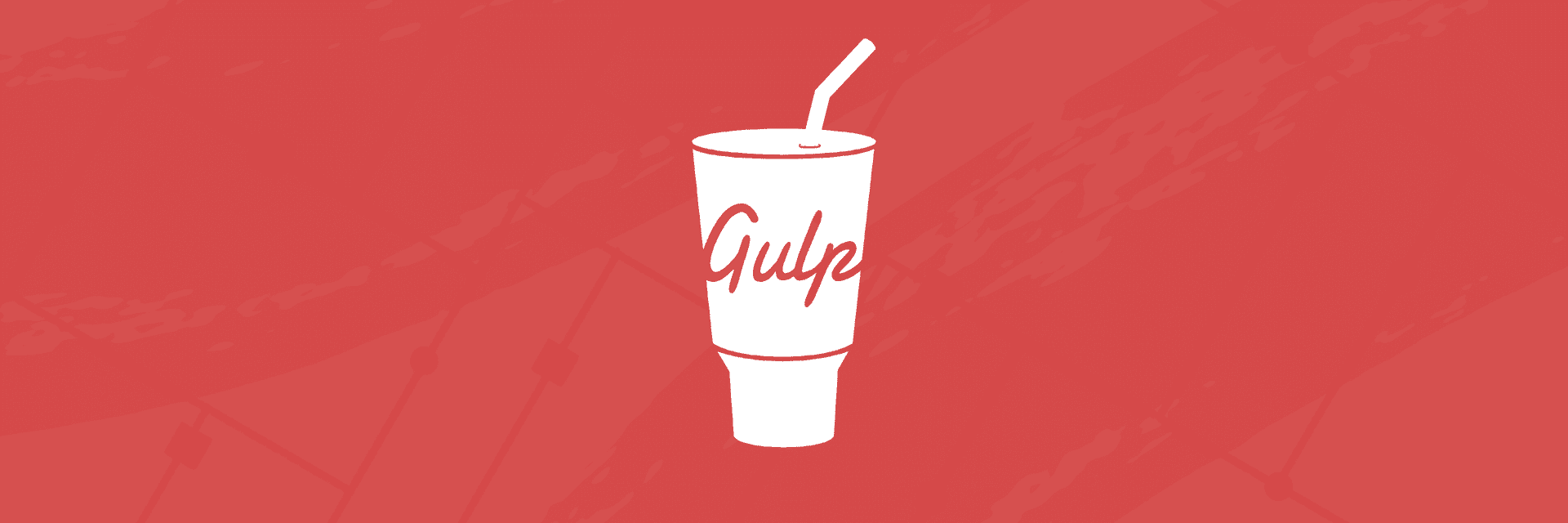
How to use Buddy to automate Gulp tasks and generate webfont from SVG files
In the recent years front-end development has evolved from simple tasks like "upload files to FTP" to complex build processes including minification of CSS/JS, expansion of SASS and LESS, and more.
These tasks can be coordinated with tools like Gulp and Grunt. Tools which help you manage the process, but on the other hand add to the complexity of the workflow. This is why we created Buddy: to help developers manage, optimize and automate their workflow, or, in other words, introduce Continuous Delivery to their projects.
Objectives of this guide
In this tutorial we'll show you how to turn SVG icons into a webfont with Gulp.js, use it with CSS in a web page, and automate the whole process with Buddy. The guide is divided into two parts:
- Part 1: Generating webfont from SVG files – where you will learn how to create webfont from SVG files using Gulp.js.
- Part 2: Automating Gulp tasks with Buddy – where you will learn how to automate the Gulp processes so that Buddy will execute your Gulp tasks every time you make a push to the repository.
Part 1: Generation of Webfont from SVG files
Prepare SVG icons
First, we need a few icons in the SVG format. You can use Illustrator, Inkscape or another vector-drawing tool. For this example I shall use icons from the Maki Icon Set.
All icons should be:
- the same size
- in solid black color
- on white background
Once you apply these settings to all icons, you are ready to create the webfont.
Image loading...
Generate package.json
In general, package.json
contains information on the npm libraries used in the project, so that you don't have to install everything manually every time you pick up the work on another computer.
To create a webfont with gulp.js, we need a package.json
with the following content:
json{ "name": "gulp-icons", "version": "1.0.0", "description": "", "scripts": { "iconfont": "gulp iconfont" }, "license": "ISC", "devDependencies": { "gulp": "^3.9.1", "gulp-consolidate": "^0.1.2", "gulp-iconfont": "^5.0.1", "underscore": "^1.8.3" } }
The main parts of our script are gulp
and gulp-iconfont
, but we will use two other dependencies, gulp-consolidate
and underscore
, to create HTML and CSS files from prepared templates. This way we'll be able to generate classes in the CSS, or variables in the SCSS.
Generate gulpfile.js
The gulpfile allows you to store Gulp commands (tasks) that let you automate your development process. In this case we need only one gulp task, because it will do everything we need:
jsvar gulp = require('gulp'), consolidate = require('gulp-consolidate'), iconfont = require('gulp-iconfont'); gulp.task('iconfont', function () { return gulp.src('iconfont-src/svg/*.svg') .pipe(iconfont({ fontName: 'iconfont', formats: ['ttf', 'eot', 'woff', 'woff2'], appendCodepoints: true, appendUnicode: false, normalize: true, fontHeight: 1000, centerHorizontally: true })) .on('glyphs', function (glyphs, options) { gulp.src('iconfont-src/iconfont.css') .pipe(consolidate('underscore', { glyphs: glyphs, fontName: options.fontName, fontDate: new Date().getTime() })) .pipe(gulp.dest('iconfont')); gulp.src('iconfont-src/index.html') .pipe(consolidate('underscore', { glyphs: glyphs, fontName: options.fontName })) .pipe(gulp.dest('iconfont')); }) .pipe(gulp.dest('iconfont')); });
How gulpfile.js works
- First we grab all SVG files from
iconfont-src/svg/
. This is the main directory where you should put all SVG sources. - Next gulp.js creates webfonts in 4 formats:
ttf
,eot
,woff
andwoff2
. You can modify this part to your desired extensions. - The rest of settings define font rendering. You can experiment with these options to your liking.
- When the font's been generated, gulp.js triggers the
glyphs
event which saves font character codepoints to CSS file and HTML demo page:iconfont-src/iconfont.css
andiconfont-src/index.html
.
Contents of iconfont-src/iconfont.css
The gulp file defines all font data. It will be used as an underscore template. The CSS template is the most important, because it will be used as the main part of our website.
You can use the icons with this syntax:
- in HTML:
<i class="icon-rocket"></i>
- in CSS:
content: '\ea09';
Remember that this is only source and all results will be placed in the iconfont
directory.
css/** <%= fontName %> Webfont */ --- font-face { font-family: "'<%= fontName %>';" --- src: url('<%= fontName %>.eot?<%= fontDate %>'); src: url('<%= fontName %>.eot?#iefix-<%= fontDate %>') format('embedded-opentype'), url('<%= fontName %>.woff2?<%= fontDate %>') format('woff2'), url('<%= fontName %>.woff?<%= fontDate %>') format('woff'), url('<%= fontName %>.ttf?<%= fontDate %>') format('truetype'); font-weight: normal; font-style: normal; } [class^='icon-']:before, [class*=' icon-']:before { font-family: '<%= fontName %>'; display: inline-block; vertical-align: middle; line-height: 1; font-weight: normal; font-style: normal; speak: none; text-decoration: inherit; font-size: inherit; text-transform: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; } /* Glyphs list */ <% _.each(glyphs, function(icon){ %> .icon-<%= icon.name %>:before { content: '\<%= icon.unicode[0].charCodeAt(0).toString(16) %>'; } <% }) %>
Contents of iconfont-src/index.html
The second template will show all icons with codepoints and CSS classes, making it easier to view the results. You don't need to create it, but it's very useful for development.
html<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <title><%= fontName %> - glyphs list</title> <link rel="stylesheet" href="iconfont.css"> <style> * { box-sizing: border-box; } body { font-family: Helvetica, Verdana, sans-serif; } .wrap { max-width: 800px; margin: 0 auto; } .glyphs-list { margin: 0; padding: 0; list-style: none } .glyphs-list li { float: left; width: 25%; padding: 16px; text-align: center; } .glyphs-list li:hover { cursor: default; background: #fafafa; } .glyphs-list li:nth-child(4n+1) { clear: left; } .glyphs-list i { font-size: 48px; line-height: 1; } .glyphs-list code { display: block; margin: 16px 0 0; } .glyphs-list input { display: block; width: 100%; margin: 16px 0 0; } </style> </head> <body> <div class="wrap"> <h1><%= fontName %> - glyphs list</h1> <ul class="glyphs-list"> <% _.each(glyphs, function(icon){ %> <li> <i class="icon-<%= icon.name %>"></i> <code>\<%= icon.unicode[0].charCodeAt(0).toString(16) %></code> <input type="text" value="icon-<%= icon.name %>"/> </li> <% }) %> </ul> </div> </body> </html>
Part 2: Automating Gulp tasks with Buddy
In this part we'll show you how to automate Gulp processes with Buddy on the basis of the previously described SVG-to-webfont task. If you haven't configured the Gulp files from the first part of this guide, you can download our sample project with the preset Gulp tasks here.
Initialize Git repository
First we need to set up a repo for your files: having your code versioned in a sound repository should be your first and most important priority as a developer. For this purpose we shall use Buddy's fully featured Git hosting with merge requests, branch management and web code editor.
In order to initialize the repo, create a new project, select Buddy as the Git provider and push your project to the repository using the URL attached:
NOTES
Alternatively, you can download our Gulp project where the files from Part 1 are already prepared and use the Import option in the right column upon creating a new project.
Buddy also supports GitHub, Bitbucket and GitLab as Git providers: just choose the service that you use when creating a new project. If you're a GitHub user, you can fork our Gulp demo project from https://github.com/buddy-works/buddy-gulp-iconfont beforehand.
Using pipelines to run Gulp tasks and deliver their results
The repo with SVG icons and config files is set: now we need to build the webfont from it and deploy the results to the server. Buddy allows you to perform these tasks using its core feature: pipelines.
Our pipeline will build the WF with Gulp and deploy it to an FTP server every time you make a commit to the repository, so that the newest version is always available at hand.
Create new pipeline
Create a new pipeline, set the trigger mode to On every push, and select Master as the target branch. This way the WF will be automatically generated every time a push to Master is made:
Image loading...(Optional) Click More options and paste the URL to your website to quickly access it from the pipeline view.
Configure Gulp action
Gulp requires Node.js to run so we need to install npm package manager first: select the Docker container with pre-installed Node.js. Image loading...
Once the action is added, enter these commands in the Execute commands field:
This Docker container with Node.js comes with `npm install -g gulp` predefined, so there's no need to install anything else.defaultnpm install gulp iconfont
Image loading...
Click Add this action to update action details.
Upload generated webfont to FTP server
Add another action and select the transfer action for your server type. In this example we'll use FTP:
Image loading...
On the action setting screen, select the Pipeline Filesystem as the source so that Buddy knows it has to deploy the fonts generated by Gulp actions along with repository files.
Provide login details to your server and set the Source path to
iconfont
. It contains all the files (we set this path ingulpfiles.js
).Confirm changes and test connection. If everything's correct, Buddy will add the action to the pipeline:
Image loading...
Test your pipeline
Congratulations! You have just automated webfont generation for your site. When you're ready, click Run pipeline > Run now to test the process. Buddy will create a Docker container, make the webfont from SVG files, and transfer it to your FTP server:
When the release is over, go to your website and check the results. All glyphs have been generated in index.html
. The page should be similar to this:
Image loading...
How to use the new webfont on your website
To use the generated WF on your site, just paste iconfont.css
in the <head>
section:
html<link rel="stylesheet" href="iconfont.css">
Now you can use custom glyphs:
- in HTML:
<i class="icon-zoo"></i>
- in CSS:
content: '\ea09';
Remember to use correct paths to your CSS!
Adding new SVG files to the generator
From now on, any time you need to add a new icon on your website just put the SVG file in the iconfont-src/scr
directory, and Buddy will take care of the rest.
Summary
This article was just an example how to use Buddy to automate your front-end processes. You can try reproducing your existing workflow onto a pipeline, for example:
- compile assets with builds and deploy them to your cloud hosting service
- run scripts on the server with SSH actions
- invite more people to your workspace and add notifications to keep them notified of the progress.
And if you ever get stuck or need advice how to get things going, drop us a line at support@buddy.works and we'll get back to you in a flash with a bag of tricks.

Jarek Dylewski
Customer Support
A journalist and an SEO specialist trying to find himself in the unforgiving world of coders. Gamer, a non-fiction literature fan and obsessive carnivore. Jarek uses his talents to convert the programming lingo into a cohesive and approachable narration.