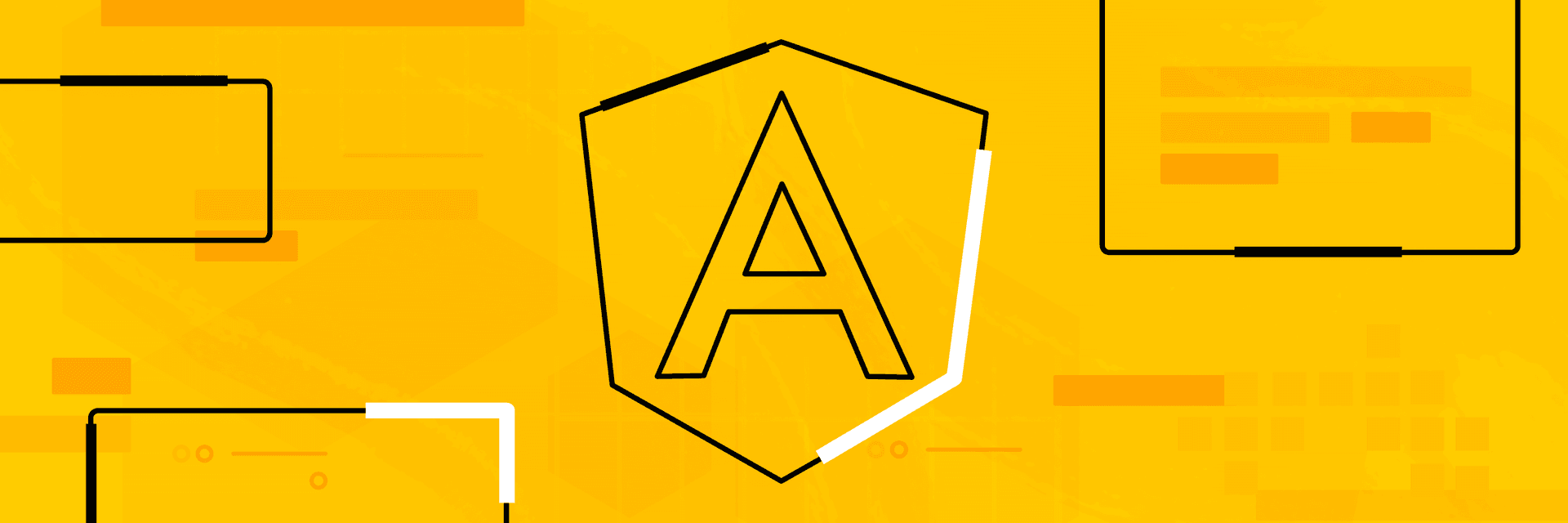
Angular Cheat Sheet for Beginners
Angular has a release-cycle of each six months which makes it seem overwhelming for a beginner to learn the Angular framework and keep up with the new changes. This is a cheat sheet that provides an overview of what it takes to learn Angular and highlights the key concepts and tools developers need to learn!
This includes Angular core concepts, and Angular CLI commands.
Intro to Angular
What is Angular?
Angular is a front-end JavaScript framework designed to create single-page applications with reusable UI components and a modular architecture.
How does it work?
Angular makes use of a new technology called Ivy which is based on an incremental DOM.
Ivy is the code name for Angular's next-generation compilation and rendering pipeline.
Starting from the Angular 9 release, the new compiler and runtime instructions are used by default instead of the older compiler and runtime, known as View Engine.
Using Angular Ivy, the compiler generates a set of template instructions. These instructions are then responsible for instantiating components, creating DOM elements, and running change detection. This is a simple figure that shows the new rendering pipeline:
Image loading...
Intro to TypeScript
Angular is written in TypeScript which is a high-level statically-typed and object-oriented language that compiles to JavaScript. It's called "JavaScript that scales" because it helps developers write maintainable and error-free code required for building large applications.
Components
Components are the basic building blocks of an Angular Application.
A component controls a part of the application screen. It's a TypeScript class that gets decorated with a @Component
decorator. You can divide your application into independent pieces that control specific parts of the app like the navigation bar.
This is an example of a component:
tsimport { Component} from '@angular/core'; @Component({ selector: 'app-navbar', template: '<header>Navbar</header>', styleUrls: ['./navbar.component.css'] }) export class NavbarComponent { title: string = "Angular Cheat Sheet"; constructor() { } }
We can invoke this compoent using the selector as follows:
html<app-navbar></app-navbar>
Generally, a component's role is enabling the user experience.
You can also use Angular Router to map components to specific routes.
A component has an associated template and one or more style sheets in plain CSS or preprocessor formats such as SCSS.
A component's template contains HTML which gets displayed when the component is activated plus some special Angular syntax called template syntax.
We can use interpolation to display values with the double curly braces {{
and }}
as delimiters. For example, we can display the value of the title
variable in our previous component in the associated template using the following syntax:
html{{title}}
Check out the docs for more template synatx.
Life-cycle events
A component has a life-cycle that begins from the moment Angular instantiates it, to when it's rendered and inserted into the DOM. But actually, this continues with watching for any changes during the life of the component using change detection mechanisms. The component's life ends when it gets destroyed and removed from the DOM.
Angular provides life-cycle hooks or methods for these key moments of the component's life:
ngOnChanges
: When an input/output binding value changes.ngOnInit
: After the firstngOnChanges
.ngDoCheck
: Developer's custom change detection.ngAfterContentInit
: After component content initialized.ngAfterContentChecked
: After every check of component content.ngAfterViewInit
: After a component's views are initialized.ngAfterViewChecked
: After every check of a component's views.ngOnDestroy
: Just before the directive is destroyed.
Services
Services are objects designed for encapsulating business logic and data that can be shared by many components. They are used to increase the reusability and modularity of apps.
A component should concern itself with presenting a part of the user interface and delegate any other concerns to services.
This is an example of service:
typescriptimport { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class DataService { constructor() { } getData(){ return [1,2,3,5]; } }
Angular has a dependency injector that helps to instantiate services and providing them to components or other services via dependency injection.
To tell Angular that a component needs a service's instance, we simply add the service to the constructor of the component as follows:
tsimport { Component} from '@angular/core'; @Component({ selector: 'app-navbar', template: '<header>Navbar</header>', styleUrls: ['./navbar.component.css'] }) export class NavbarComponent { constructor(private dataService: DataService) { } }
We have injected DataService
in NavbarComponent
which gives the component access to the service class.
Modules
Angular has a modular architecture and makes use of NgModules for achieving that.
Angular apps have at least one NgModule class, which is referred to asthe root module. By convention, it's named AppModule
and resides in the src/app/app.module.ts
file.
Angular CLI
Angular CLI is the official utility for initializing and working with Angular projects. It allows developers to quickly get up and running without going through all the hassles required for setting up a modern front-end environment for Angular development.
Before you can use Angular CLI, you need to have Node.js and npm (Node package manager) installed on your development machine. If that’s not the case, you can install Node.js using one of the following methods:
- Grab the installer for your operating system from the official website.
- Use the official package manager for your system.
- Use a Node version manager such as NVM. This is the most recommended way!
Head over to a new command-line interface and run the following command:
bash$ npm install -g @angular/cli
$$
Note: If your system demands that you prepend sudo to your command in macOS or Linux systems, or use a Command prompt with administrator access in Windows to install Angular CLI globally, you simply need to fix your npm permissions. Please refer to the official npm website for instructions, or simply, as we previously recommended, install a version manager like NVM which takes care of all of the required configurations.
The previous command will install angular/cli v12.0.0 (the current version at the time of this writing).
You can also use npx to run Angular CLI from npm without installing it:
bash$ npx -p @angular/cli ng new angularapp
$$
After installing the tool, we can generate a new Angular 12 project using the following command:
bash$ ng new angularapp
$$
You’ll be asked for the following information:
- Would you like to add Angular routing? (y/N) y.
- Which stylesheet format would you like to use? (Use arrow keys), pick CSS.
You can also provide options as follows:
bash$ ng new angularapp --verbose=true --skipTests=true --skipGit=true --style=css --routing=true
$$
- --verbose=true: This tells Angular to output more log information to the console while starting, generating the necessary artifacts, and installing the packages.
- --skipTests=true: This tells Angular CLI to skip generating test files.
- --skipGit=true: Skip initializing a git repository for the project.
- --routing=true: This tells the CLI to generate a routing module for our application and set up routing.
- --style=css: Sets the CSS preprocessor for styling.
When Angular CLI generates the project’s files and install the needed dependencies, you can navigate to your project’s folder and start a live-reload development server using the following commands:
bash$ cd angularapp $ ng serve
$$$
Angular live development server is listening on localhost:4200
, open your browser on http://localhost:4200/
to see your application up and running!
App Structure
A new Angular project will have the following folder structure.
Image loading...
Let's briefly go through them one by one.
package.json
: this contains the dependencies and the scripts to start the development server, run tests, and build production bundles. This is required by any Node.js project.node_modules
: this folder is where your npm dependencies are installed./src
folder: where most development will take place.src/main.ts
: bootstraps the Angular app.src/app/app.component.ts
: contains the rootApp
component.src/app/app.module.ts
: contains the root application module.src/index.html
: the file that's served from the server that invokes the root component and bootstraps the Angular application.src/styles.css
: this file contains the global styles of the app.angular.json
: the configuration file for your project. 10.tsconfig.json
: the configuration file for the TypeScript compiler.src/environments/
: this folder contains the build configuration for the target environments.src/assets/
: this folder contains static assets such as images.
In the main.ts
we can find the entry point of our app. It makes use of Angular’s JIT compiler to compile the app code and then bootstraps the app’s root module which exists in the app/app.module.ts
file.
Inside the app/
folder, we have the root component of our application which has the app-root
selector used to invoke and render the components in its children in the main src/index.html
file:
html<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>Angularapp</title> <base href="/"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="icon" type="image/x-icon" href="favicon.ico"> </head> <body> <app-root></app-root> </body> </html>
This file is the main HTML file that gets served when your visit the app in the web browser.
You can generate various artifacts using the ng generate
command. For example, let's generate a component named navbar;
bash$ ng generate component navbar
$$
This will create the following files:
src/app/navbar/navbar.component.css
src/app/navbar/navbar.component.html
src/app/navbar/navbar.component.ts
It will also update the src/app/app.module.ts
file by importing the component into the root module as follows:
tsimport { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { NavbarComponent } from './navbar/navbar.component'; @NgModule({ declarations: [ AppComponent, NavbarComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
If you have more than one module and want to import the component into another module other than the root module (default), you simply need to specify the module as follows:
bash$ ng generate component navbar --module core
$$
Here we suppose that we have created a core module using the following command:
bash$ ng generate module core
$$
You can also generate services as follows:
bash$ ng generate service data
$$
This will create a src/app/data.service.ts
file with the following code:
tsimport { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class DataService { constructor() { } }
You can find more commands from the official website.
More to Learn!
That's it for this Angular Cheat Sheet for beginners. Of course, this is just a very concise article so it cannot cover every single aspect of Angular. But I do hope it has been a great introduction to at least help anyone embark on an Angular journey without feeling intimidated or too overwhelmed.